Decision Making Statement in C
Decision Making Statement in C
Decision making statement is depending on the condition block need to be executed or not which is decided by condition.
If the condition is "true" statement block will be executed, if condition is "false" then statement block will not be executed.
In this section we are discuss about if-then (if), if-then-else (if else), and switch statement. In C language there are three types of decision making statement.
- if
- if-else
- switch
if-then Statement
if-then is most basic statement of Decision making statement. It tells to program to execute a certain part of code only if particular condition is true.
Syntax
if(condition) { ....... ....... }
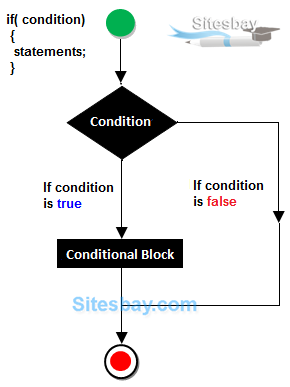
- Constructing the body of "if" statement is always optional, Create the body when we are having multiple statements.
- For a single statement, it is not required to specify the body.
- If the body is not specified, then automatically condition part will be terminated with next semicolon ( ; ).
else
It is a keyword, by using this keyword we can create a alternative block for "if" part. Using else is always optional i.e, it is recommended to use when we are having alternate block of condition.
In any program among if and else only one block will be executed. When if condition is false then else part will be executed, if part is executed then automatically else part will be ignored.
if-else statement
In general it can be used to execute one block of statement among two blocks, in C language if and else are the keyword in C.
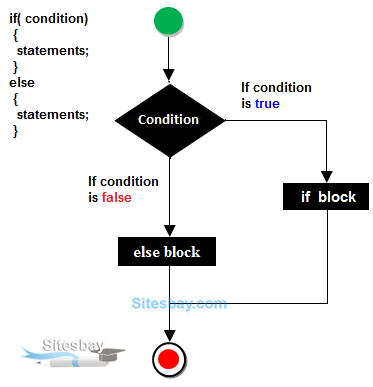
Syntax
if(condition) { ........ statements ........ } else { ........ statements ........ }
In the above syntax whenever condition is true all the if block statement are executed remaining statement of the program by neglecting else block statement. If the condition is false else block statement remaining statement of the program are executed by neglecting if block statements.
Example
#include<stdio.h> #include<conio.h> void main() { int time=10; clrscr(); if(time>12) { printf("Good morning"); } { printf("Good after noon"); } getch(); }
Output
Good morning
Switch Statement
A switch statement work with byte, short, char and int primitive data type, it also works with enumerated types and string.
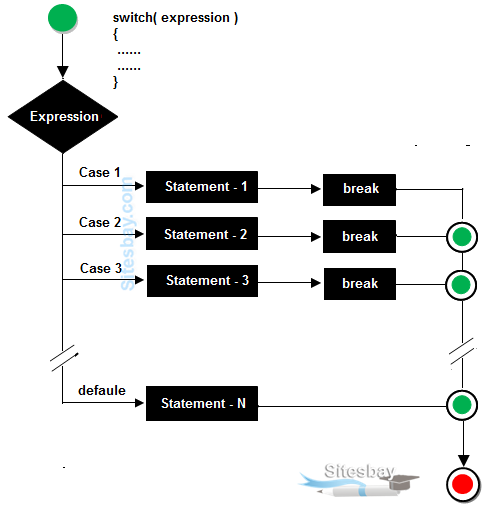
Syntax
switch(expression/variable) { case value: //statements // any number of case statements break; //optional default: //optional //statements }
Rules for apply switch
- With switch statement use only byte, short, int, char data type.
- You can use any number of case statements within a switch.
- Value for a case must be same as the variable in switch .
Limitations of switch
Logical operators cannot be used with switch statement. For instance
Example
case k>=20: //is not allowed
Switch case variables can have only int and char data type. So float data type is not allowed.
Syntax
switch(ch) { case1: statement 1; break; case2: statement 2; break; }
In this ch can be integer or char and cannot be float or any other data type.
Example of Switch case
#include<stdio.h> #include<conio.h> void main() { int ch; clrscr(); printf("Enter any number (1 to 7)"); scanf("%d",&ch); switch(ch) { case 1: printf("Today is Monday"); break; case 2: printf("Today is Tuesday"); break; case 3: printf("Today is Wednesday"); break; case 4: printf("Today is Thursday"); break; case 5: printf("Today is Friday"); break; case 6: printf("Today is Saturday"); break; case 7: printf("Today is Sunday"); break; default: printf("Only enter value 1 to 7"); } getch(); }
Output
Enter any number (1 to 7): 5 Today is Friday
Note: In switch statement default is optional but when we use this in switch default is executed at last whenever all cases are not satisfied the condition.