Armstrong Program in C++
Armstrong Number Program in C++
Armstrong number is a number that is the sum of its own digits each raised to the power of the number of digits is equal to the number itself. Some Armstrong numbers is 0, 1, 153, 370, 371, 407, 1634 etc.
For write this code we need some basic concept about If Else Statement in C++ and While Loop concept in C++
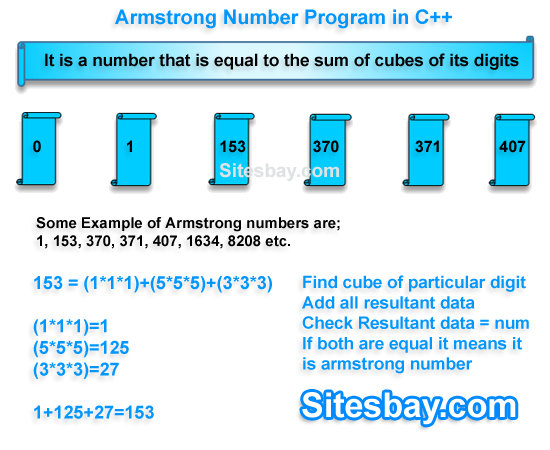
For example:
Three Digits Armstrong number is 153, 1 ^ 3 + 5 ^ 3 + 3 ^ 3 = 153
Four Digits Armstrong number is 1634, 1 ^ 4 + 6 ^ 4 + 3 ^ 4 + 4 ^ 4 + = 1634
Let's try to understand why 153 is an Armstrong number.
Example
153 = (1*1*1)+(5*5*5)+(3*3*3) where: (1*1*1)=1 (5*5*5)=125 (3*3*3)=27 So: 1+125+27=153
Let's try to understand why 371 is an Armstrong number.
Example
371 = (3*3*3)+(7*7*7)+(1*1*1) where: (3*3*3)=27 (7*7*7)=343 (1*1*1)=1 So: 27+343+1=371
Let's try to understand why 1634 is an Armstrong number.
Example
1634 = (1*1*1*1)+(6*6*6*6)+(3*3*3*3)+(4*4*4*4) where: (1*1*1*1) = 1 (6*6*6*6) = 1296 (3*3*3*3) = 81 (4*4*4*4) = 256 So: 1+1296+81+256= 1634
3 Digits Armstrong Program in C++
Armstrong Number Program in C++
#include<iostream.h> #include<conio.h> void main() { int arm=0,a,b,c,d,no; clrscr(); cout<<"Enter any num: "; cin>>no; d=no; while(no>0) { a=no%10; no=no/10; arm=arm+a*a*a; } if(arm==d) { cout<<"Armstrong"; } else { cout<<"not Armstrong"; } getch(); }
Output
Enter any number: 23 not Armstrong
Any number of Digits Armstrong Program in C++
Check Number is Armstrong or not in C++
#include<iostream.h> #include<conio.h> void main() { int num; int f,rem,sum=0,temp,a=0; clrscr(); cout<<"Enter any number: "; cin>>num; temp=num; while(temp != 0) { temp=temp/10; a=a+1; } f=num; while(f!=0) { rem=f%10; sum = sum + power(rem,a); f=f/10; } if( sum == num ) cout<<"\n Armstrong number "; else cout<<"\n Not a Armstrong number"; getch(); } int power(int c, int d) { int pow=1; int i=1; while(i<=d) { pow=pow*c; i++; } return pow; }
Output
Enter any number: 153 Armstrong number
Explanation of Program
temp=num; while(temp != 0) { temp=temp/10; a=a+1; }
This code is for calculate the number of digits in given/entered number by user. above temp variable assign the enter number by user (temp=num). Every time temp divided by 10 and result store in temp if temp become zero loop will be terminated