Convert Decimal to Hexadecimal Program in C++
Advertisements
C++ program to Convert Decimal to Hexadecimal
To convert binary to hexadecimal in C++ Program, we have to ask to the user to enter any number in binary to convert it into hexadecimal, then display the equivalent hexadecimal value on the screen.
Binary Numbers: Binary number Base is 2 because Binary numbers is 0 and 1. Examples of Decimal Number is 110, 111, 1011, 1111, 1010 etc
Hexadecimal Numbers: Hexadecimal number Base is 16 because Hexadecimal represent value of 0 to 9 and A to F. Examples of Hexadecimal Number is 1E, F, 3E8, 5A... etc.
Example
Input Binary Number : 1011 Euivalent Hexadecimal Number : B Input Binary Number : 11011 Euivalent Hexadecimal Number : 1B Input Binary Number : 11110 Euivalent Hexadecimal Number : 1E
Algorithom to Convert Binary to Hexadecimal
- Divide the number by 16.
- Get the integer quotient for the next iteration.
- Get the remainder for the hex digit.
- Repeat the steps until the quotient is equal to 0.
C++ program to convert Binary to Hexadecimal
#include<iostream.h> #include<conio.h> #define 1000 void main() { char binnum[MAX], hexa[MAX]; int temp; long int i=0,j=0; clrscr(); cout<<"Enter any Binary Number: "; cin>>binnum; while(binnum[i]) { binnum[i] = binnum[i] -48; ++i; } --i; while(i-2>=0) { temp = binnum[i-3] *8 + binnum[i-2] *4 + binnum[i-1] *2 + binnum[i] ; if(temp > 9) hexa[j++] = temp + 55; else hexa[j++] = temp + 48; i=i-4; } if(i ==1) hexa[j] = binnum[i-1] *2 + binnum[i] + 48 ; else if(i==0) hexa[j] = binnum[i] + 48 ; else --j; cout<<"Equivalent Hexadecimal Number is: \n"; while(j>=0) { cout<<hexa[j--]; } getch(); }
Output
Enter any Binary Number : 10011 Equivalent Hexadecimal Number is: 13
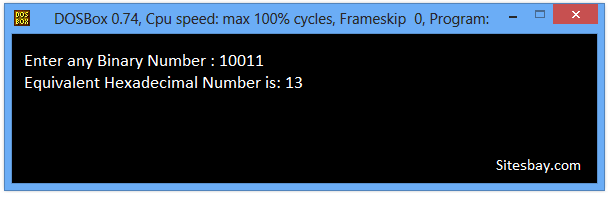
Google Advertisment