Print Star Pattern in Java
Java Program to Print star pattern
In Java language you can print triangle shape using for loop and also using while loop, Here we discuss about how to print Triangle of stats in very simple and easy way. But before learning this topic first read carefully if, if..else, for loop and while loop concept in detail. To Print Star Pattern in Java you need looping concept, if..else statement and print() and println() function.
To print patterns of numbers and stars (*) in Java Programming, we need to use two loops, first is outer loop and the second is inner loop. Where outer loop is responsible for print rows and the inner loop is responsible for print columns.
Java pattern program enhances the coding skill, logic, and looping concepts. It is mostly asked in Java interview to check the logic and thinking of the programmer. We can print a Java pattern program in different designs. To learn the pattern program, we must have a deep knowledge of the Java loop, such as for loop do-while loop. In this section, we will learn how to print a pattern in Java. We have classified the Java pattern program into three categories;
- Start Pattern
- Number Pattern
- Character Pattern
Whenever you design logic for a pattern program, first draw that pattern in the blocks, as we have shown in the following image. The figure presents a clear look of the pattern.
Each pattern program has two or more than two loops. The number of the loop depends on the complexity of pattern or logic. The first for loop works for the row and the second loop works for the column. In the pattern programs, Java for loop is widely used.
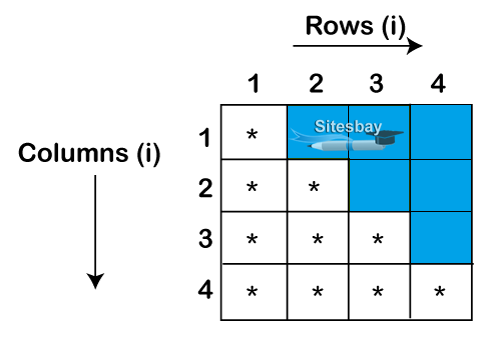
Difference Between print() and println()
- System.out.print("....."): is used for display message on screen or console but cursor don't move in new line.
- System.out.println("....."): is used for display message on screen or console and cursor move in new line.
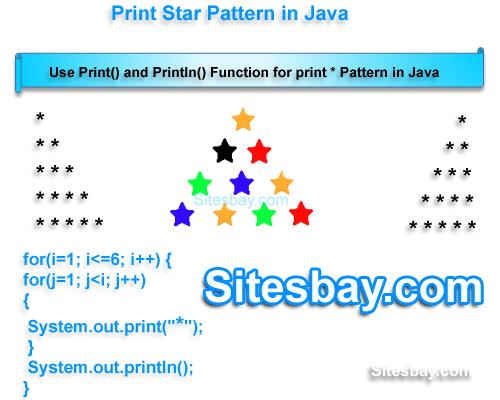
Print Star Pattern in Java
class Star { public static void main(String[] args) { int i; for(i=5;i>=1;i--) { System.out.println("*"); } } }
Output
* * * * *
Syntax to compile and run java program
Syntax
for compile -> c:/>javac Star.java for run -> c:/>java Star
Code Explanation: Here we use println() function to print output, after print one output cursor move in new line. The main difference between print() and println() function is; print() function don't move cursor in new line after print output on screen but in case of println() function it move cursor in new line after print output.
Print Star Pattern in Java
class Star { public static void main(String[] args) { int i,j,k; for(i=1; i<=4; i++) { for(j=1; j<=5; j++) { System.out.print("*"); } System.out.println(" "); } } }
Output
***** ***** ***** *****
Print star pattern in java
class StarTriangle { public static void main(String[] args) { int i,j,k; for(i=1; i<=5; i++) { for(j=4; j>=i; j--) { System.out.print(" "); } for(k=1; k<=(2*i-1); k++) { System.out.print("*"); } System.out.println(""); } } }
Output
* * * * * * * * * * * * * * *
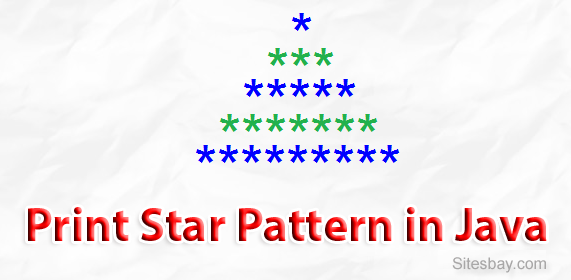
How to Make Triangle in Java
class Star { public static void main(String[] args) { int i, j, k; for(i=5;i>=1;i--) { for(j=5;j>i;j--) { System.out.print(" "); } for(k=1;k<(i*2);k++) { System.out.print("*"); } System.out.println(); } } }
Output
* * * * * * * * * * * * * * * * * * * * * * * * *
Print Star Pattern in Java
class Star { public static void main(String[] args) { int i,j; for(i=1; i<=6; i++) { for(j=1; j<i; j++) { System.out.print("*"); } System.out.println(); } } }
Output
* * * * * * * * * * * * * * *
Print * Triangle in Java
class StarPattern { public static void main(String args[]) { int i, j, k=1; for(i=0; i<5; i++) { for(j=0; j<k; j++) { System.out.print("* "); } k = k + 2; System.out.println(); } } }
Output
* * * * * * * * * * * * * * * * * * * * * * * * *
Print Simple Pattern in Java
class Star { public static void main(String[] args) { int i, j; for(i=5;i>=1;i--) { for(j=1;j<=i;j++) { System.out.print("*"); } System.out.println(); } } }
Output
* * * * * * * * * * * * * * *
Print Triangle of Stars in Java
class Star { public static void main(String[] args) { int i, j, k; for(i=5;igt;=1;i--) { for(j=1;jlt;i;j++) { System.out.print(" "); } for(k=5;k>=i;k--) { System.out.print("*"); } System.out.println(); } } }
Output
* * * * * * * * * * * * * * *
Print Star Pattern in Java
class Star { public static void main(String[] args) { int i, j, k; for(i=5;i>=1;i--) { for(j=5;j>i;j--) { System.out.print(" "); } for(k=1;k<=i;k++) { System.out.print("*"); } System.out.println(); } } }
Output
* * * * * * * * * * * * * * *
Print * Pattern in Java
class Star { public static void main(String[] args) { int i, j, k; for(i=1;i<=5;i++) { for(j=i;j<5;j++) { System.out.print(" "); } for(k=1;k<(i*2);k++) { System.out.print("*"); } System.out.println(); } for(i=4;i>=1;i--) { for(j=5;j>i;j--) { System.out.print(" "); } for(k=1;k<(i*2);k++) { System.out.print("*"); } System.out.println(); } } }
Output
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *