Awt Layout Management
Advertisements
Awt Layout Management
Layout is a logical container used to arrange the GUI component in proper order within the frame, in java.awt package some of the layout is existing an predefined classes as shown below;
- FlowLayout
- BoarderLayout
- GridLayout
- GridbagLayout
- CardLayout
FlowLayout
This layout is used to arrange the GUI components in a sequential flow (that means one after another in horizontal way)
You can also set flow layout of components like flow from left, flow from right.
FlowLayout Left
Frame f=new Frame(); f.setLayout(new FlowLayout(FlowLayout.LEFT));
FlowLayout Right
Frame f=new Frame(); f.setLayout(new FlowLayout(FlowLayout.RIGHT));
Example of FlowLayout
import java.awt.*; class FlowLayoutDemo { public static void main(String[] args) { Frame f=new Frame(); f.setTitle("myframe"); f.setBackground(Color.cyan); f.setForeground(Color.red); f.setLayout(new FlowLayout(FlowLayout.LEFT)); Button b1=new Button("Submit"); Button b2=new Button("Cancel"); f.add(b1); f.add(b2); f.setSize(500,300); f.setVisible(true); } }
Download code Click
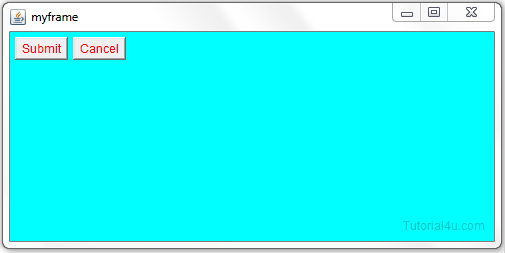
BoarderLayout
This layout is used to arrange the GUI components in S directions of frame as shown below.
Example
import java.awt.*; class BorderDemo { public static void main(String[] args) { Frame f=new Frame(); f.setTitle("myframe"); f.setSize(500,400); f.setBackground(Color.white); f.setLayout(new BorderLayout()); Button b1=new Button("first"); b1.setBackground(Color.green); b1.setForeground(Color.white); Button b2=new Button("second"); b2.setBackground(Color.yellow); b2.setForeground(Color.black); Button b3=new Button("third"); b3.setBackground(Color.red); b3.setForeground(Color.white); Button b4=new Button("fourth"); b4.setBackground(Color.red); b4.setForeground(Color.white); /*Button b5=new Button("center"); b5.setBackground(Color.cyan); b5.setForeground(Color.white);*/ f.add("North",b1); f.add("South",b2); f.add("East",b3); f.add("West",b4); //f.add(b5); f.setVisible(true); } }
Download code Click
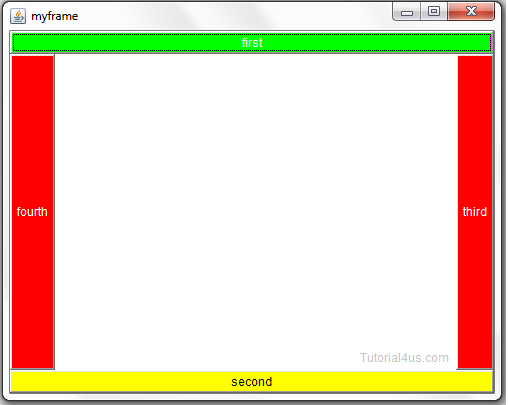
GridLayout
This layout is used to arrange the GUI components in the table format.
Example of GridLayout
import java.awt.*; class Grid extends Frame { Grid() { Frame f=new Frame(); f.setTitle("myframe"); f.setSize(500,400); f.setBackground(Color.white); f.setLayout(new GridLayout(2,2)); Button b1=new Button("one"); b1.setBackground(Color.green); b1.setForeground(Color.white); Button b2=new Button("two"); b2.setBackground(Color.yellow); b2.setForeground(Color.black); Button b3=new Button("three"); b3.setBackground(Color.red); b3.setForeground(Color.white); Button b4=new Button("four"); b4.setBackground(Color.cyan); b4.setForeground(Color.white); /*Button b5=new Button("center"); b5.setBackground(Color.cyan); b5.setForeground(Color.white);*/ f.add("one",b1); f.add("two",b2); f.add("three",b3); f.add("four",b4); //f.add(b5); f.setVisible(true); } }; class GridDemo { public static void main(String [] args) { Grid g1=new Grid(); } }
Download code Click
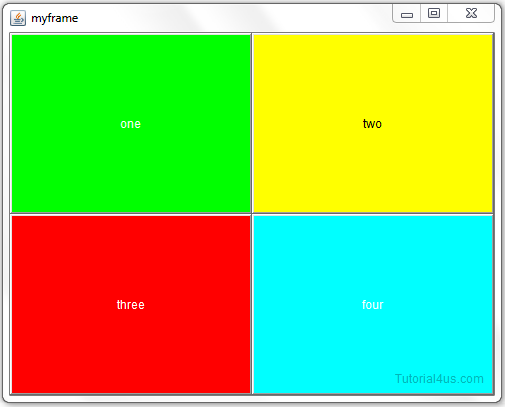
CardLayout
This layout is used to arrange multiple cards within the same frame.
Syntax
Frame f=new Frame(); f.setLayout(new CardLayout());
GridbagLayout
This layout is used to arrange the GUI components in un-order table structured.
Google Advertisment