Queue Interface in Java
Advertisements
Queue Interface in Java
Queue Interface defines the queue data structure, which stores elements in the order in which they are inserted. New additions go to the end of the line, and elements are removed from the front. It creates a first-in first-out system.
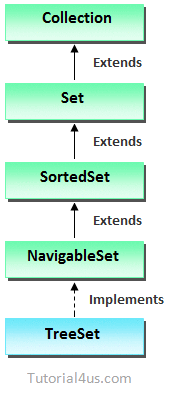
Methods of Queue Interface
- public boolean add(object)
- public boolean offer(object)
- public remove()
- public poll()
- public element()
- public peek()
Queue Interface can be implement using following classes;
- PriorityQueue
- ArrayDeque
PriorityQueue
Example of PriorityQueue
import java.util.*; class PriorityQueueDemo { public static void main(String args[]) { PriorityQueue<String> queue=new PriorityQueue<String>(); // Add Elements queue.add("Deo"); queue.add("Smith"); queue.add("Piter"); queue.add("Poter"); System.out.println("Iterating the queue Elements:"); Iterator itr=queue.iterator(); while(itr.hasNext()){ System.out.println(itr.next()); } } }
Output
Iterating the queue Elements: Deo Smith Piter Porte
PriorityQueue
Example of PriorityQueue
import java.util.*; class PriorityQueueDemo { public static void main(String args[]) { PriorityQueue<String> queue=new PriorityQueue<String>(); // Add Elements queue.add("Deo"); queue.add("Smith"); queue.add("Piter"); queue.add("Poter"); // Removing Elements queue.remove(); queue.poll(); System.out.println("Removing the queue Elements:"); Iterator itr=queue.iterator(); while(itr.hasNext()){ System.out.println(itr.next()); } } }
Output
Removing the queue Elements: Piter Porte
Google Advertisment