Steps to Write JDBC Program
Steps to write JDBC Program
There are 6 steps to connect any java application with the database using JDBC. They are as follows:
- Load the JDBC driver class or register the JDBC driver.
- Establish the connection
- Create a statement
- Execute the sql commands on database and get the result
- Print the result
- Close the connection
1. Register the driver class
In this step we load the JDBC driver class into JVM. This step is also called as registering the JDBC driver. The forName() method of Class class is used to register the driver class. This method is used to dynamically load the driver class. This step can be completed in two ways.
- class.forName("fully qualified classname")
- DriveManager.registerDriver(object of driver class)
Syntax of forName() method
public static void forName(String className)throws ClassNotFoundException
Sun.Jdbc.Odbc.JdbcOdbcDriver is a driver class provided by Sun MicroSystem and it can be loaded into jvm like the following.
Syntax
class.forName("Sun.Jdbc.Odbc.JdbcOdbcDriver");
Syntax
Sun.Jdbc.Odbc.JdbcOdbcDriver jod=new Sun.Jdbc.Odbc.JdbcOdbcDriver(); DriverManager.registerDriver(jod);
2. Create the connection object
In this step connection between a java program and a database will be opened. To open the connection, we call getConnection() method of DriverManager class.
For getConnection() method we need to pass three parameters.
- url
- username
- password
url: url is used to select one register JDBC driver among multiple registered driver by DriverManager class.
username and password: username and password are used for authentication purpose.
Syntax of getConnection() method
1) public static Connection getConnection(String url)throws SQLException 2) public static Connection getConnection(String url,String name,String password) throws SQLException
Example to establish connection with the Oracle database
Connection con=new DriverManager.getConnection(url, username, password); Example: Connection con=new DriverManager.getConnection(Jdbc:Odbc:< dsn >", "scott","tiger");
3. Create the Statement object
To transfer sql commands from java program to database we need statement object. To create a statement object we call createStatement() method of connection interface. The createStatement() method of Connection interface is used to create statement. The object of statement is responsible to execute queries with the database.
Syntax of createStatement() method
public Statement createStatement()throws SQLException
Example to create the statement object
Statement stmt=new createStatement();
4. Executing queries
Call any one of the following three methods of Statement interface is used to execute queries to the database and to get the output.
- executeUpdate(): Used for non-select operations.
- executequery(): Used for select operation.
- execute(): Used for both select or non-select operation.
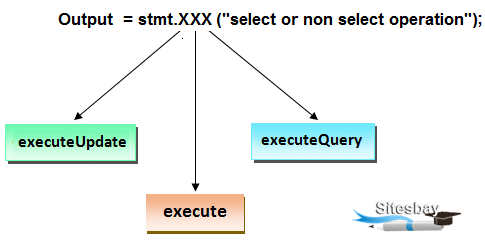
5. Print the result.
Syntax
System.out.println(output);
6.Closing connection : Close the connection.
By closing connection object statement and ResultSet will be closed automatically. The close() method of Connection interface is used to close the connection.
Syntax of close() method
Syntax of close() method
public void close()throws SQLException
Example for close connection
con.close();
Example
import java.sql.*; class CreateTable { public static void main(String[] args) throws Exception { //step-1 Class.forName("sun.jdbc.odbc.JdbcOdbcDriver"); System.out.println("driver is laoded"); //step-2 Connection con=DriverManager.getConnection("jdbc:odbc:ramadsn","system","system"); System.out.println("connection is established"); //step-3 Statement stmt=con.createStatement(); System.out.println("statement object is cretaed"); //step-4 int i=stmt.executeUpdate("create table student(sid number(3),sname varchar2(10),marks number(5))"); //step-5 System.out.println("Result is="+i); System.out.println("table is created"); //step-6 stmt.close(); con .close(); } }