Control Flow Statement
Control Flow Statements in Java
The Control Flow Statements are used to control the flow of execution of the program. In Java programming language there are three types of control flow statement available.
- Decision making or selection statement (if, if-else, switch)
- Looping or Iteration statement (while, for, do-while)
- Breaking or Jumping statement (break, continue)
Decision Making Statements
Decision making statement statements is also called selection statement. That is depending on the condition block need to be executed or not while is decided by condition. If the condition is "true" statement block will be executed, if condition is "false" then statement block will not be executed.
In this section we are discuss about if-then, if-then-else, and switch statement. In java there are three types of decision making statement.
- if
- if-else
- switch
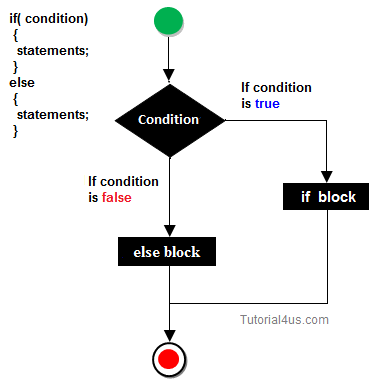
if-then Statement
if-then is the most basic statement of the decision making statement. It tells to program to execute a certain part of code only if a particular condition or test is true.
Syntax
if(condition) { Statement(s) }
- Constructing the body if always optional, that is recommended to create the body when we are having multiple statements.
- For a single statement, it is not required to specify the body.
- If the body is not specified, then automatically condition parts will be terminated with next semicolon ;.
Else
- It is a keyword, by using this keyword we can create an alternative block for "if" part.
- Using else is always optional i.e, it is recommended to use when we are having alternate block of condition.
- When we are working with if else among those two block at any given point of time only one block will be executed.
- When if condition is false, then else part will be executed, if part is executed, then automatically else part will be ignored.
if-else statement
In general, it can be used to execute one block of statement among two blocks, in Java language if and else are the keyword in Java.
Syntax
if(condition) { Statement(s) } else { Statement(s) } Statement(s)
In the above syntax whenever the condition is true all the if block statement are executed remaining statement of the program by neglecting else block statement If the condition is false else block statement remaining statement of the program are executed by neglecting if block statements.
A Java program to find the addition of two numbers if first number Is greater than the second number Otherwise find the subtraction of two numbers.
Example
import java.util.*; class num { public static void main(String args[]) { int c; System.out.println("Enter any two num"); Scanner sn=new Scanner(System.in); int a=sn.next(); int b=sn.next(); if(a>b) { c=a+b; } else { c=a-b; } System.out.println("Result="+c); } }
Switch Statement
A switch statement work with byte, short, char and int primitive data type, it also works with enumerated types and string.
Syntax
switch(expression/variable) { case value: //statements // any number of case statements break; //optional default: //optional //statements }
Rules for apply switch statement
With switch statement use only byte, short, int, char data type. You can use any number of case statements within a switch. The value for a case must be same as the variable in a switch.
Limitations of switch statement
Logical operators cannot be used with a switch statement. For instance
Example
case k>=20:
is not allowed
Switch case variables can have only int and char data type. So float data type is not allowed. For instance in the switch syntax given below:
Syntax
switch(ch) { case 1: statement-1; break; case 2: statement-2; break; }
In this ch can be integer or char and cannot be float or any other data type.
Looping statement
These are the statements execute one or more statement repeatedly a several number of times. In Java programming language there are three types of loops are available, that is, while, for and do-while.
Advantage with looping statement
- Length on the developer is reducing.
- Burden on the developer is reducing.
- Burden of memory space is reduced time consuming process to execute the program is reduced.
Difference between conditional and looping statement
Conditional statement executes only once in the program were as looping statement executes repeatedly several numbers of time.
While loop
- When we are working with while loop always pre-checking process will be occurred.
- Pre-checking process means before the evolution of statement block condition parts will be executed.
- While loop will be repeated in clockwise direction.
Syntax
while(condition) { Statement(s) Increment / decrements (++ or --); }
Example
class whileDemo { public static void main(String args[]) { int i=0; while(i<10) { System.out.println(+i); i++; }
Output
1 2 3 4 5 6 7 8 9 10
for loop
for loop is a statement which allows code to be repeatedly executed. For loop contains 3 parts.
- Initialization
- Condition
- Increment or Decrements
Syntax
for ( initialization; condition; increment ) { statement(s); }
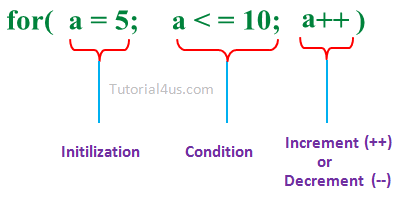
- Initialization: step is executed first and this is executed only once when we are entering into the loop first time. This step allows to declare and initialize any loop control variables.
- Condition: is the next step after initialization step, if it is true, the body of the loop is executed. If it is false, the body of the loop does not execute and flow of control goes outside the for loop.
- Increment or Decrements: After completion of Initialization and Condition steps loop body code is executed and then Increment or Decrements steps is executed. This statement allows to update any loop control variables.
Flow Diagram
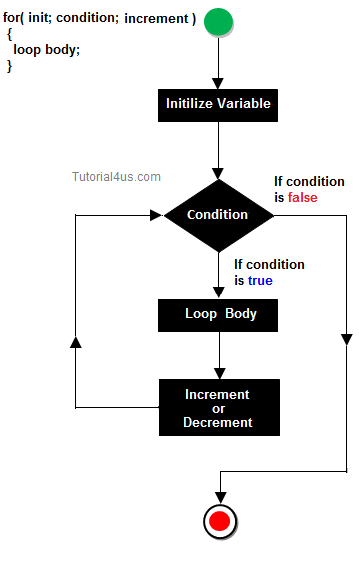
Control flow of for loop
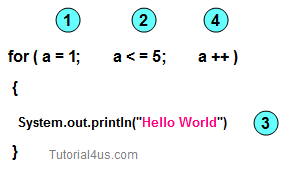
- First Initialize the variable
- In second step check condition
- In third step control goes inside loop body and execute.
- At last increase the value of variable
- Same process is repeat until condition not false.
A java program to display any message exactly 10 times.
Example
class hello { public static void main(String args[]) { int i; for (i=0: i<10; i++) { System.out.println("Hello Friends"); } } }
do-while
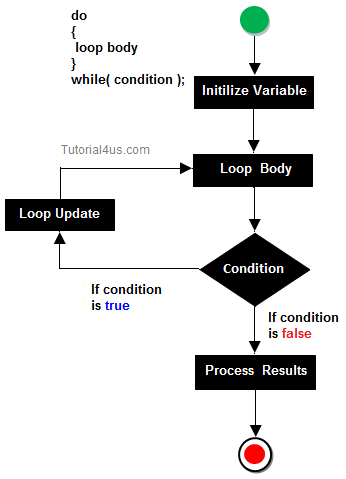
- when we need to repeat the statement block at least 1 then go for do-while.
- In do-while loop post-checking process will be occur, that is after execution of the statement block condition part will be executed.
Syntax
do { Statement(s) increment/decrement (++ or --) }while();
Example
class dowhileDemo { public static void main(String args[]) { int i=0; do { System.out.println(+i); i++; } While(i<10); } }
Outpet
1 2 3 4 5 6 7 8 9 10
Read more about these things in separate
- Static variable in Java
- Static method in Java
- Static block in Java
- Difference between Static and non-static method in Java
- Difference between Static and non-static method in Java
- Difference Between Static and non-Static Variable in Java