String Compare in Java
Advertisements
String Compare in Java
There are three way to compare string object in java:
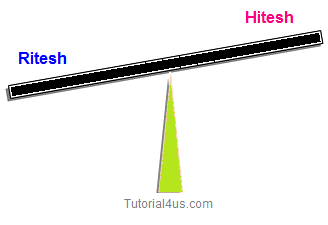
- By equals() method
- By == operator
- By compreTo() method
equals() Method in Java
equals() method always used to comparing contents of both source and destination String. It return true if both string are same in meaning and case otherwise it returns false. It is case sensitive method.
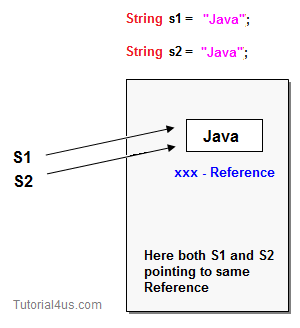
Example
class StringHandling { public static void main(String arg[]) { String s1="Hitesh"; String s2="Raddy"; String s3="Hitesh"; System.out.println("Compare String: "+s1.equals(s2)); System.out.println("Compare String: "+s1.equals(s3)); } }
Output
Compare String: false Compare String: true
== or Double Equals to Operator in Java
== Operator is always used for comparing references of both source and destination objects but not their contents.
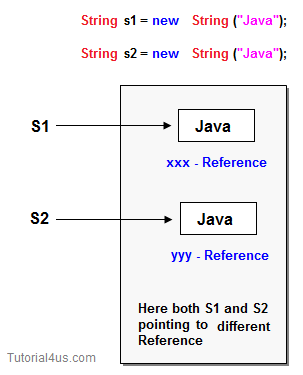
Example
class StringHandling { public static void main(String arg[]) { String s1=new String("java"); String s2=new String("java"); if(s1==s2) { System.out.println("Strings are same"); } else { System.out.println("Strings are not same"); } } }
Output
Strings are not same
compareTo() Method in Java
comapreTo() method can be used to compare two string by taking Unicode values. It returns 0 if the string are same otherwise returns either +ve or -ve integer.
Example
class StringHandling { public static void main(String arg[]) { String s1="Hitesh"; String s2="Raddy"; int i; i=s1.compareTo(s2); if(i==0) { System.out.println("Strings are same"); } else { System.out.println("Strings are not same"); } } }
Output
Strings are not same
Difference between equals() method and == operator
equals() method always used to comparing contents of both source and destination String.
== Operator is always used for comparing references of both source and destination objects but not their contents.
Google Advertisment